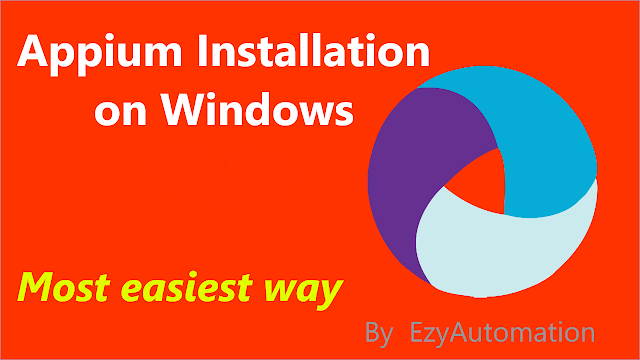
Prepare Windows PC for Automating your Android Application by using APPIUM and run your first Automation script with TestNG framework. In this tutorial series I'm trying to show how to automat all types of mobile app (Native, Hybrid, and Web).
Here I'm following few simple steps for the installation process.
This tutorial not for those who are expert in Automation/APPIUM
Prerequisite to use APPIUM
1. ANDROID SDK
2. JDK (Java Development Kit)This tutorial not for those who are expert in Automation/APPIUM
1. ANDROID SDK
3. APPIUM server for Windows
4. IntelliJ IDEA (Community Edition)
Steps:
Step 1: Download all the prerequisite
Step 2: Install JDK and set environment variable path
Step 3: Install IntelliJ IDEA
Step 4: Install SDK and set environment variable path
4. IntelliJ IDEA (Community Edition)
Steps:
Step 1: Download all the prerequisite
Step 2: Install JDK and set environment variable path
Step 3: Install IntelliJ IDEA
Step 4: Install SDK and set environment variable path
Step 5: Install APPIUM server
Step 6: Run your first Automation script
Step 1: Download all the prerequisiteStep 6: Run your first Automation script
- ANDROID SDK (Download link)
- JDK (Java Development Kit) (Download link)
- APPIUM For Windows (Download link)
- IntelliJ IDEA (Community Edition) (Download link)
Step 2: Install JDK and set Environment variable path
- Install JDK
- My Computer/This PC > Right button click > Properties > Advanced system settings > Environment Variables (below the Advanced tab) > New (below the System variables) > Enter Variable name(JAVA_HOME) > Enter Variable value(Eg: )
- Double click on 'Path'(Below the System variables) > New > Enter environment variable (%JAVA_HOME%\bin) > OK
- Open command prompt > type java -version > Enter > Java version should be appeared like below:
- Java installation and path setting is Done
Step 3: Install IntelliJ IDEA
Step 4: Install SDK and set Environment variable path
- Keep and extract the sdk in any drive of your PC
- Enter sdk folder > Click on ‘SDK Manager’ to open
- Copy sdk folder path (Eg: C:\sdk) > My Computer/This PC > Right button click > Properties > Advanced system settings > Environment Variables (below the Advanced tab) > New (Below the System variables) > Enter Variable name(ANDROID_HOME) > Enter Variable value(C:\sdk) > OK > Double click on 'Path'(below the System variables) > New > Enter environment variable (%ANDROID_HOME%) > OK
- Double click on 'Path'(Below the System variables) > New > Enter environment variable (C:\sdk\platform-tools) > OK
- Double click on 'Path'(Below the System variables) > New > Enter environment variable (C:\sdk\tools) > OK
- Open command prompt > type adb> Enter > Android Debug Bridge version should be appeared like below
- SDK Installation and Environment variable path settings are Done
Step 6: Run your first Automation script
- Open IntelliJ IDEA > Create a new project
- Select Maven > Browse java directory as Project SDK > Next
- Enter GroupId and ArtifactId> Next
- Enter Project name > Finish
- Open pom.xml file
- Add below maven dependencies in 'pom.xml' file
<dependencies>
<dependency>
<groupId>io.appium</groupId>
<artifactId>java-client</artifactId>
<version>5.0.4</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.7.1</version>
</dependency>
</dependencies>
If you want to get the updated version of above three dependencies please go the links below- Enable auto import and wait until downloaded all dependencies
- Install the test app(which application you want to test) on device, in my case I have installed Calculator app on my device.
- Collect appPackage by using uiautomatorviewer
- Install 'APK Info' app on your device from play store (Play Store link), for collecting appActivity
- Go to Project > src > main > test > java > right click > Create a java class(CalculatorTest.java) > Copy the below code and Paste in the class
import io.appium.java_client.android.AndroidDriver; import org.openqa.selenium.By; import org.openqa.selenium.remote.DesiredCapabilities; import org.testng.Assert; import org.testng.annotations.AfterTest; import org.testng.annotations.BeforeTest; import org.testng.annotations.Test; import java.net.MalformedURLException; import java.net.URL; public class CalculatorTest { AndroidDriver driver; @BeforeTest public void androidSetup() throws MalformedURLException { DesiredCapabilities dc = new DesiredCapabilities(); dc.setCapability("platformVersion", "6.0"); dc.setCapability("deviceName", "Android"); dc.setCapability("appPackage", "com.google.android.calculator"); dc.setCapability("appActivity", "com.android.calculator2.CalculatorGoogle"); driver = new AndroidDriver(new URL("http://localhost:4723/wd/hub"), dc); } @Test(priority = 0) public void addOperationTest() throws InterruptedException { Thread.sleep(2000); driver.findElement(By.id("digit_7")).click(); driver.findElement(By.id("op_add")).click(); driver.findElement(By.id("digit_8")).click(); driver.findElement(By.id("eq")).click(); String actualResult = driver.findElement(By.id("result")).getText(); Assert.assertEquals(actualResult, "15"); System.out.println("Add operation: pass"); } @Test(priority = 1) public void subOperationTest() throws InterruptedException { Thread.sleep(2000); driver.findElement(By.id("digit_8")).click(); driver.findElement(By.id("op_sub")).click(); driver.findElement(By.id("digit_7")).click(); driver.findElement(By.id("eq")).click(); String actualResult = driver.findElement(By.id("result")).getText(); Assert.assertEquals(actualResult, "1"); System.out.println("Sub operation: pass"); } @AfterTest public void tearDown(){ driver.quit(); } }
- Run Appium server
- Connect your Android device with PC
- Right click on class > Run
Enjoy the fun of automation ...... :)
Comments
Post a Comment